View on Github View on Youtube

Python Beginner
How to Build the Viral Word Game WORDLE
Original Game: Wordle - A daily word game
Introduction
In this tutorial I am going to walk through how to build a simple Wordle game in your terminal using Python. The final product will look like this:
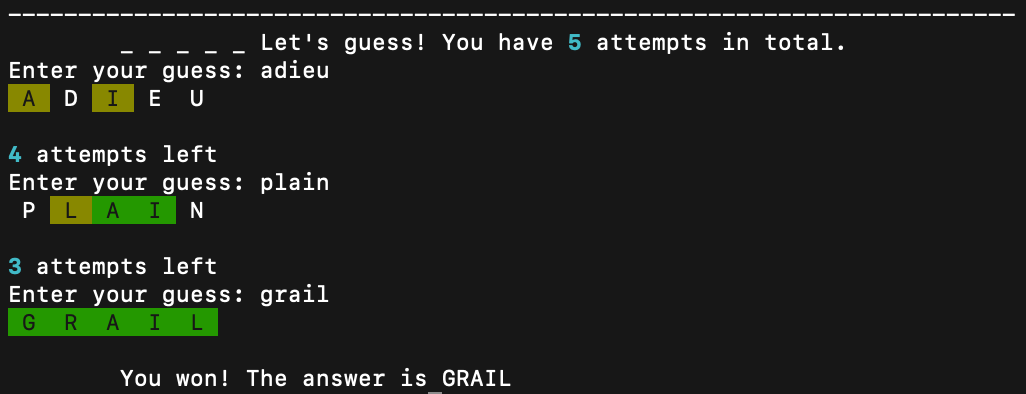
Implementation
1. Visualization
Rich (https://github.com/Textualize/rich)
Since the terminal is not so friendly for visualization, I imported Rich, a Python library for rich text and beautiful formatting in the terminal.
Installation guide can be found in the above link.
For this project only the print function from Rich is needed.
It can print reverse text with customed background colours.
Syntax:
from rich import print
#Print inverted gray A :
print('[reverse gray] A [reverse gray]')
#Print inverted yellow B :
print('[reverse yellow] B [reverse yellow]')
#Print inverted green C :
print('[reverse green] c [reverse green]')
2. Get an answer from a word list
This first thing to do is to get an answer from a list of 5-letter words. It’s easy to download a 5-letter-word list online. Here’s an example. You can also copy all and paste it into a new .txt file.
Next, you should read
the .txt file and store the words into a Python list, then pick one word from random
to be your answer.
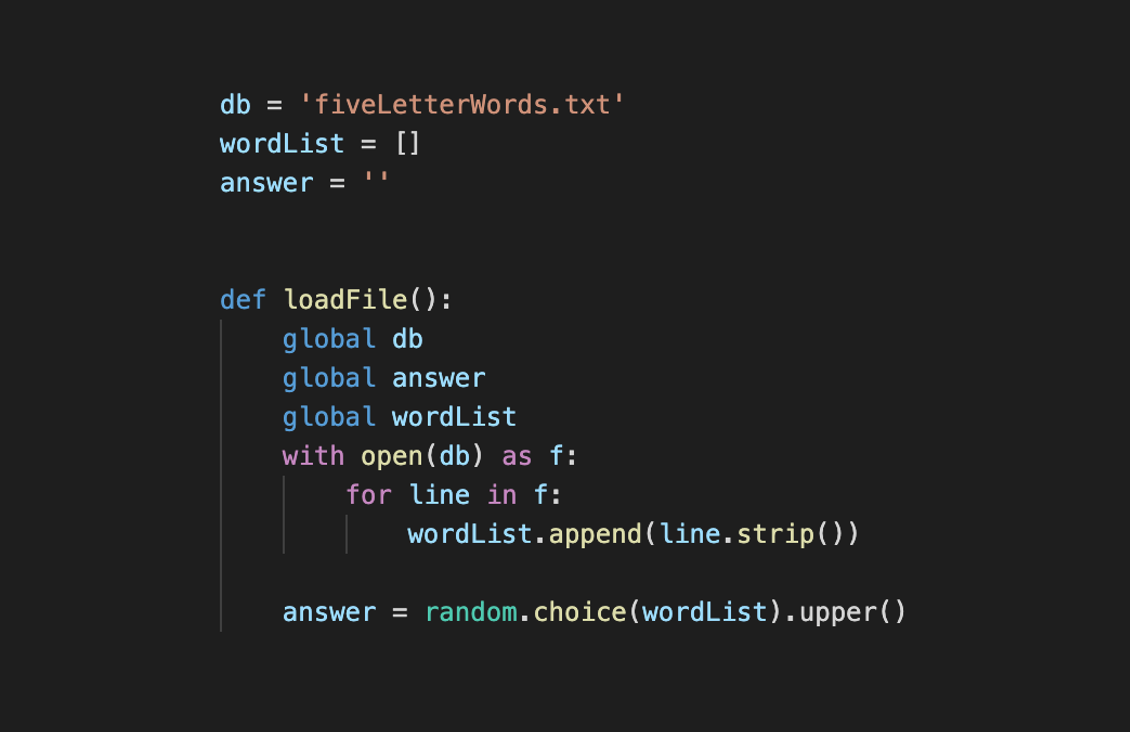
try:
loadFile()
except:
print('OOOPS! No such file called \'{0}\' in your directory!'.format(db))
sys.exit()
3. Play
After getting an answer, you can start playing.
In the Beginning you would like to initiate a variable maxAttempt
to be a fixed integer, e.g. 5.
Then the game is going to loop for maximum 5 times. The only two ways to break the loop is either (1) the user get the correct answer or (2) the user has used up all 5 chances.
Initially you should initiate the following variables:
isWin
= False
currentAttempt
= 0
maxAttempt
= 5
welcomeMessage
= '_ _ _ _ _ Let\'s play Wordle!'
Pseudocode
while currentAttempt
is less than maxAttempt
:
if (it is the first attempt):
print the welcomeMessage
else:
print how many attempts left
# Handle user input
isValid
= False (set the user input to not valid)
while (the input
is NOT valid):
keep asking user for input
check if the input
is valid, if yes change isValid
to True
# Display coloured hint
for every letter
in the user input
:
if (the letter
is in the right place):
print the letter
inverted green
elif (the letter
exists somewhere in the answer
):
print the letter
inverted yellow
else:
print the letter
inverted gray
# Check if has won
if the user input
is exactly the same with the answer
:
turn isWin
to True
break the while loop
currentAttempt
+= 1
Note: Uppercase-Lowercase Handling
Uppercase and lowercase letters are different characters in Computer Science. When your answer
is 'HELLO'
but the user input
is 'hello'
, the computer won't consider it correct.
Therefore, it is better to convert all strings to uppercase for easier comparsion.
You can use .upper()
function.
Handle user input
First, convert the input to uppercase.
Second, check if the input meets the following requirements:
- the input has and only has precisely 5 characters
- all characters are alphabets
- the case-converted input is an existing word (it can be checked by seeing if the word exists in your word list)
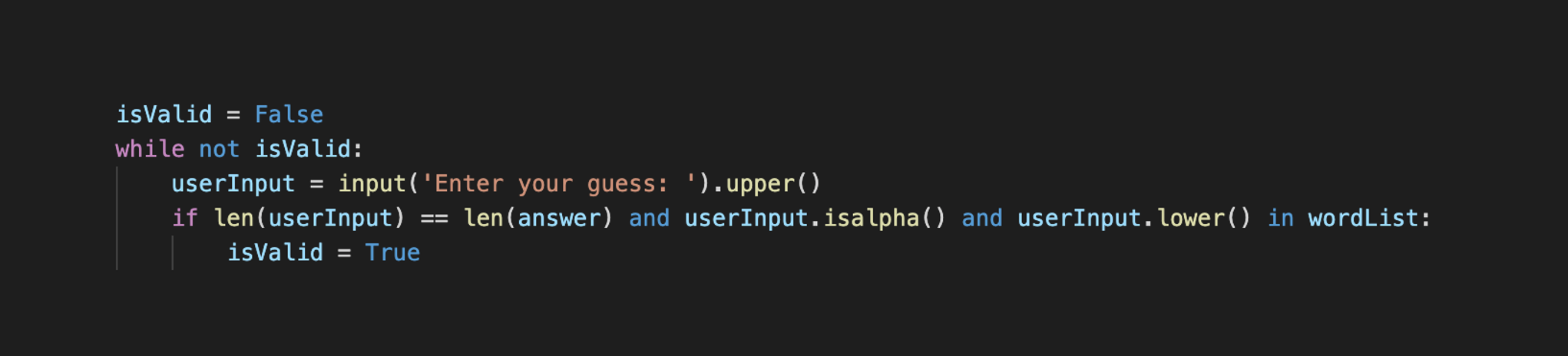
Display coloured hint
As mentioned in previous part (Visualization) we will use a Python library called Rich to display the hints in three colours.
To be precise, we will loop through, every time, the valid input from user to check letter by letter.
For example, suppose the answer
is 'MAPLE' and the user input
is 'FLAME'.
You loop through 'FLAME' to get the hint colour.
- F: not in 'MAPLE' → F
- L: in 'MAPLE' but not in right position → L
- A: in 'MAPLE' but not in right position → A
- M: in 'MAPLE' but not in right position →   M
- E: in the right place → E
Code
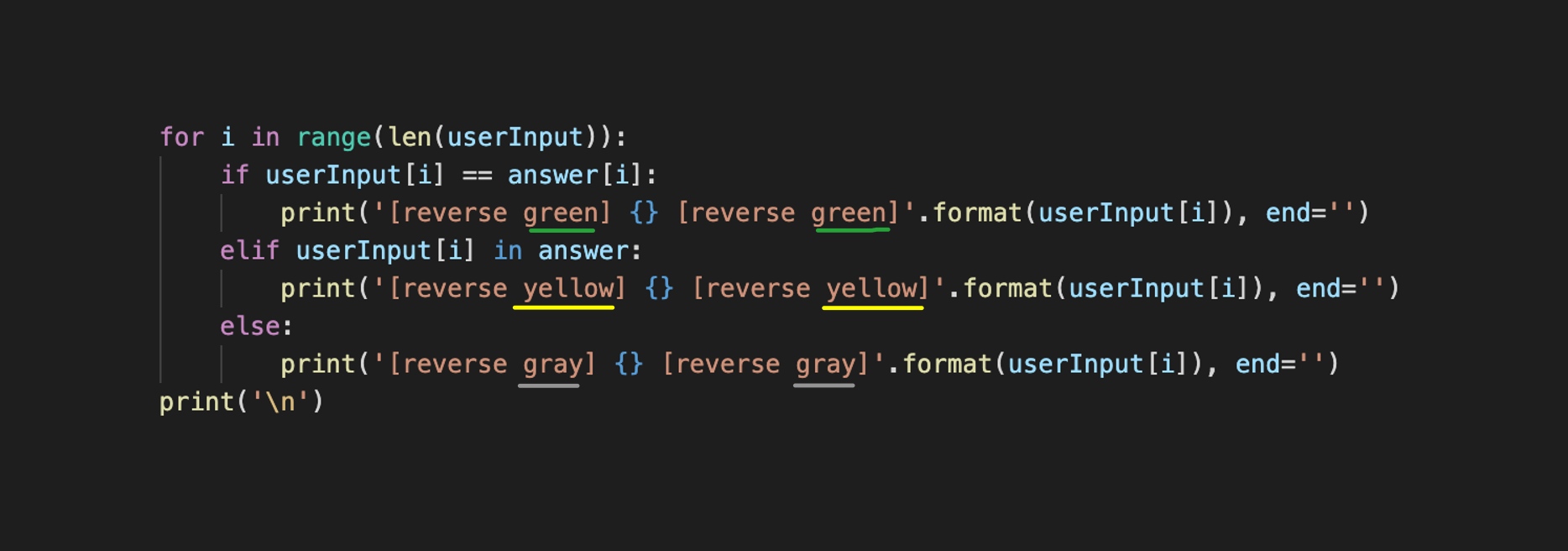
Check if has Won
Code
The last part is relatively simple. Just check if the user has got the answer completely correct.
(Also, all 5 letters would be shown green to the user.)
If they do, turn isWin
to True and break the while loop.
isWin
has no use in the play
function, but it can be used and changed outside the function because I set it to be a global variable.
When the user has done playing, we are going to a new function to print the result
.
If isWin
= True, we will print the winning message.
Contrarily, we will print the losing message, as well as the correct answer.
Conclusion
That's all for building the simple word guessing game. I hope you all enjoy!
Demo on Youtube
You've just finished reading: How to Build the Viral Word Game WORDLE